Transforming PySpark DataFrame String Column to Array for Explode Function
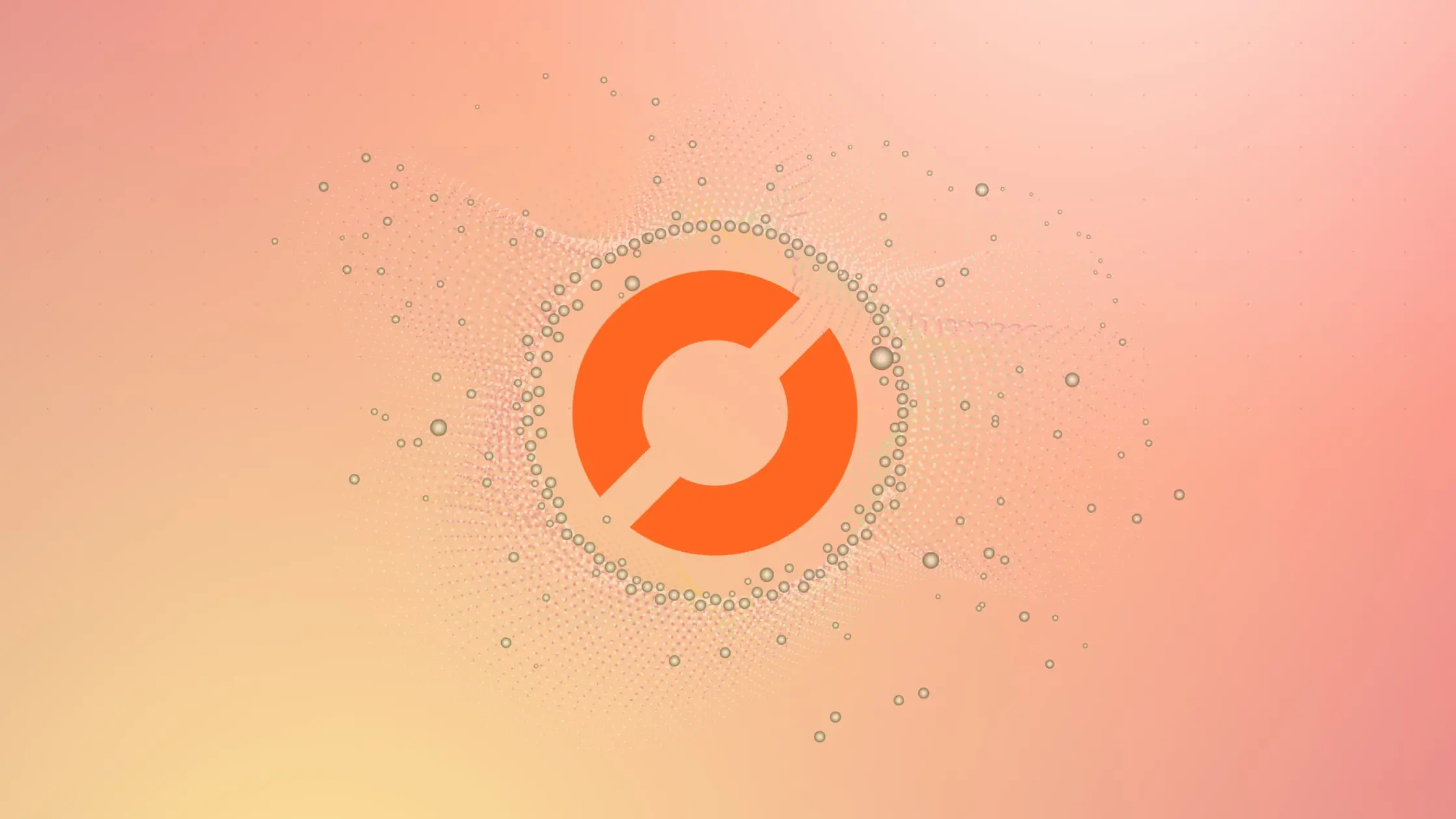
Table of Contents
- What is PySpark?
- Why Change a Column from String to Array?
- Step-by-Step Guide to Transforming String Column to Array
- Handling Common Errors
- Conclusion
In the world of big data, PySpark has emerged as a powerful tool for data processing and analysis. One of the most common tasks data scientists encounter is manipulating data structures to fit their needs. In this blog post, we’ll explore how to change a PySpark DataFrame column from string to array before using the explode function.
What is PySpark?
PySpark is the Python library for Apache Spark, an open-source, distributed computing system used for big data processing and analytics. PySpark allows data scientists to write Spark applications using Python APIs, making it a popular choice for handling large datasets.
Why Change a Column from String to Array?
In PySpark, the explode
function is used to transform each element of an array in a DataFrame column into a separate row. However, this function requires the column to be an array. If your data is in string format, you’ll need to convert it to an array before using explode
.
Step-by-Step Guide to Transforming String Column to Array
Let’s walk through the process of transforming a string column to an array in a PySpark DataFrame.
Step 1: Import Necessary Libraries
First, we need to import the necessary libraries. We’ll need PySpark and its submodules.
from pyspark.sql import SparkSession
from pyspark.sql.functions import split
Step 2: Initialize SparkSession
Next, we initialize a SparkSession, which is the entry point to any PySpark functionality.
spark = SparkSession.builder.appName('pyspark-by-examples').getOrCreate()
Step 3: Create a DataFrame
For this example, let’s create a DataFrame with a single string column.
data = [("James,John", "USA"), ("Robert,Michael", "Canada")]
df = spark.createDataFrame(data, ["Names", "Country"])
df.show()
Output:
+--------------+-------+
| Names|Country|
+--------------+-------+
| James,John| USA|
|Robert,Michael| Canada|
+--------------+-------+
Step 4: Convert String Column to Array
Now, we’ll use the split
function to convert the string column into an array. The split
function takes two arguments: the name of the column to split and the delimiter.
df = df.withColumn("Names", split(df["Names"], ","))
df.show()
Output:
+-----------------+-------+
| Names|Country|
+-----------------+-------+
| [James, John]| USA|
|[Robert, Michael]| Canada|
+-----------------+-------+
Step 5: Use Explode Function
Finally, we can use the explode
function on the array column.
from pyspark.sql.functions import explode
df = df.select(explode(df.Names).alias("Name"), df.Country)
df.show()
Output:
+-------+-------+
| Name|Country|
+-------+-------+
| James| USA|
| John| USA|
| Robert| Canada|
|Michael| Canada|
+-------+-------+
Handling Common Errors
Null Values
Suppose your DataFrame has null values in the name
column. To handle these null values before applying the transformation, you can use the na.fill
function to replace them with an empty string. Here’s an example:
from pyspark.sql import SparkSession
from pyspark.sql import functions as F
# Create a Spark session
spark = SparkSession.builder.appName("example").getOrCreate()
# Example DataFrame with null values
df_with_nulls = spark.createDataFrame([(1, "apple,orange"),
(2, None),
(3, "grape,banana,kiwi")],
["id", "name"])
# Handling null values and transforming string column to array
df_transformed_with_nulls = df_with_nulls.na.fill("") \
.withColumn("data_array", F.split(F.col("name"), ","))
df_transformed_with_nulls.show(truncate=False)
Output:
+---+-----------------+---------------------+
|id |data_str |data_array |
+---+-----------------+---------------------+
|1 |apple,orange |[apple, orange] |
|2 | |[] |
|3 |grape,banana,kiwi|[grape, banana, kiwi]|
+---+-----------------+---------------------+
In this example, we use the na.fill("")
function to replace null values with an empty string before applying the split function.
Trimming Whitespaces:
If your string elements have leading or trailing whitespaces, it’s advisable to trim them to avoid inconsistencies.
from pyspark.sql.functions import split, trim, col
# Example DataFrame with whitespaces
df_with_whitespaces = spark.createDataFrame([(1, " apple , orange "),
(2, " grape, banana, kiwi "),
(3, "pear, pineapple")],
["id", "data_str"])
# Trimming whitespaces and transforming string column to array
df_transformed_with_whitespaces = df_with_whitespaces \
.withColumn("data_str", trim(col("data_str"))) \
.withColumn("data_array", split(col("data_str"), ","))
df_transformed_with_whitespaces.show(truncate=False)
Output:
+---+-------------------+-----------------------+
|id |data_str |data_array |
+---+-------------------+-----------------------+
|1 |apple ; orange |[apple ; orange] |
|2 |grape, banana, kiwi|[grape, banana, kiwi]|
|3 |pear, pineapple |[pear, pineapple] |
+---+-------------------+-----------------------+
In this example, the trim
function is used to remove leading and trailing whitespaces from the data_str
column before applying the split
function.
By addressing these common errors in your PySpark DataFrame transformations, you ensure more robust and accurate results, especially when working with string columns that may have missing values or inconsistent formatting.
Conclusion
Transforming a string column to an array in PySpark is a straightforward process. By using the split
function, we can easily convert a string column into an array and then use the explode
function to transform each element of the array into a separate row. This technique is particularly useful when dealing with large datasets where manual data manipulation is not feasible.
Remember, PySpark is a powerful tool for data processing and analysis. Understanding its functions and how to manipulate data structures in PySpark is crucial for any data scientist working with big data.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.