Python Pandas Dataframe How to Multiply Entire Column with a Scalar
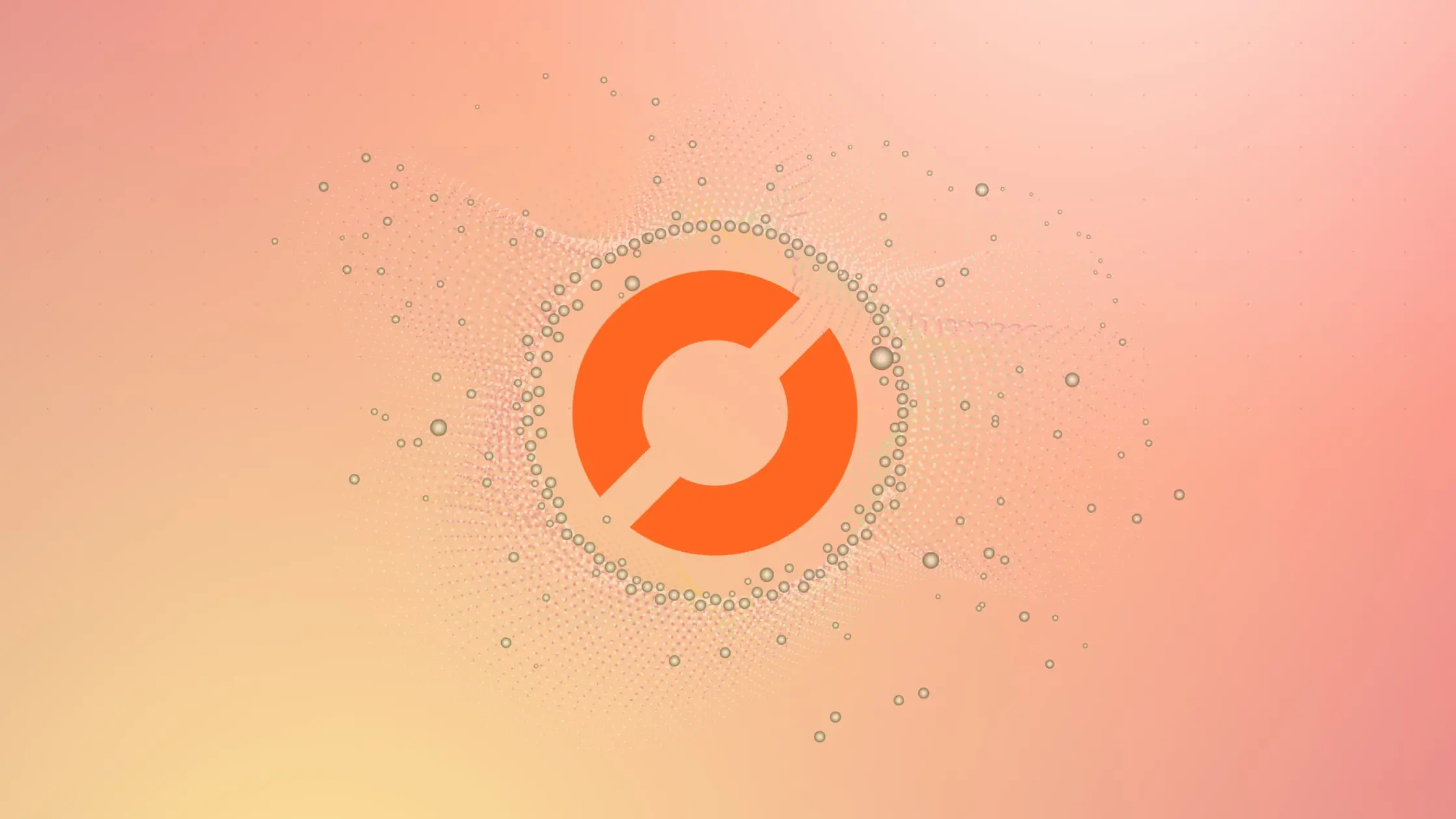
As a data scientist or software engineer, working with large datasets is a common occurrence. One of the most widely used libraries for data manipulation in Python is Pandas. Pandas provides a powerful and easy-to-use data structure called Dataframe that allows us to work with tabular data efficiently. In this article, we will discuss how to multiply an entire column of a Pandas Dataframe with a scalar.
What is a Pandas Dataframe?
A Pandas Dataframe is a 2-dimensional labeled data structure with columns of potentially different types. It is similar to a spreadsheet or a SQL table, but with more powerful features. A Dataframe can be created from various data sources such as CSV, Excel, SQL database, or even from a Python dictionary.
Multiplying an Entire Column with a Scalar
Sometimes, it is necessary to multiply an entire column of a Pandas Dataframe with a scalar. For example, suppose we have a dataset that contains the sales revenue of a company for the last 5 years, and we want to increase the revenue by 10% for all the years. In this case, we can use the Pandas Dataframe’s multiply()
method to achieve this.
The multiply()
method multiplies the values of two Dataframes or a Dataframe and a scalar. To multiply an entire column of a Dataframe with a scalar, we can easily use *
or we can the column name and the scalar to the multiply()
method. Let’s see an example.
import pandas as pd
# create a sample dataframe
df = pd.DataFrame({
'Year': [2016, 2017, 2018, 2019, 2020],
'Revenue': [10000, 12000, 15000, 18000, 22000]
})
# multiply the 'Revenue' column with 1.1 (10% increase)
df['Revenue_with_*'] = df['Revenue'] * 1.1
df['Revenue_with_mul'] = df['Revenue'].multiply(1.1)
# print the updated dataframe
print(df)
Output:
Year Revenue Revenue_with_* Revenue_with_mul
0 2016 10000 11000.0 11000.0
1 2017 12000 13200.0 13200.0
2 2018 15000 16500.0 16500.0
3 2019 18000 19800.0 19800.0
4 2020 22000 24200.0 24200.0
In the above example, we first created a sample Dataframe that contains the sales revenue of a company for the last 5 years. We then multiplied the Revenue
column with 1.1 using 2 different ways, which increased the revenue by 10%. Finally, we printed the updated Dataframe.
Conclusion
In this article, we discussed how to multiply an entire column of a Pandas Dataframe with a scalar. We simply used *
and the Pandas Dataframe’s multiply()
method to achieve this. Multiplying an entire column of a Dataframe with a scalar is a common operation in data manipulation and can be useful in various scenarios. Pandas provides easy and efficient ways to perform this operation.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.